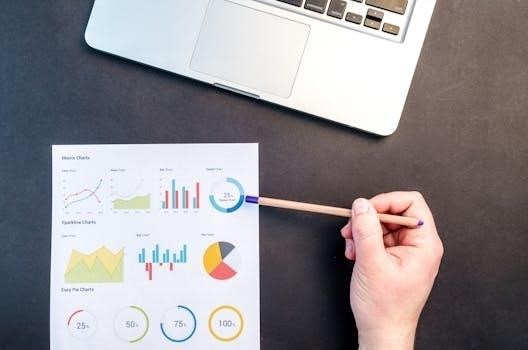
STD File Formats and Their Uses
STD files serve various purposes, including vector graphics templates, where they act as a basis for creating consistent designs. They are also used in semiconductor testing as a proprietary file format known as STDF. These files facilitate the storage of analysis results and data.
STD Files in Vector Graphics
In the realm of vector graphics, STD files function primarily as templates, not as complete images themselves. These template files, often created using drawing programs like Apache OpenOffice Draw, contain default drawing elements. This allows designers to create multiple vector images with a consistent look and feel, streamlining the design process. The STD file provides a foundation upon which new images are built, ensuring uniformity across various projects. Rather than starting from scratch each time, users can utilize these templates, modifying and expanding upon them as needed. This method is particularly useful for maintaining brand consistency or for projects requiring similar visual elements. The use of STD templates enhances efficiency and promotes a standardized visual language within design workflows, acting as a time-saving tool for repetitive design tasks.
STD Files as Templates
STD files frequently act as templates across different applications, providing a standardized base for generating new documents or graphics. These templates, often created within specific software, contain pre-defined elements, layouts, or structures that users can adapt for their own purposes. For instance, in drawing programs like Apache OpenOffice Draw, STD files serve as foundations for creating new vector images, ensuring a consistent style and feel. Similarly, in other contexts, STD templates can provide pre-set formatting and data structures for various types of documents. This templating mechanism speeds up production processes and guarantees uniformity by providing a starting point that eliminates the need to start from scratch each time. Users can easily modify and build upon these templates to suit their unique needs, making them a versatile tool for efficiency and consistency. The use of STD files as templates reduces manual effort.
STD Files in Semiconductor Testing (STDF)
In the semiconductor industry, the STD file extension often refers to the Standard Test Data Format (STDF), a proprietary file format initially developed by Teradyne. However, STDF has become an industry-wide standard for storing semiconductor test information, serving as the primary output from automatic test equipment (ATE) platforms. These files contain a detailed record of the testing process and the results obtained from various semiconductor devices. STDF files are essential for tracking and analyzing the performance of these devices, enabling engineers to identify issues and make informed decisions about production quality. Due to its widespread use, many software tools and platforms support the importing and analysis of STDF files, which promotes interoperability in the semiconductor supply chain. This format ensures data integrity and ease of accessibility for all those involved in the semiconductor manufacturing process. The STDF files play a critical role in quality control.
Working with STD Files
Working with STD files involves various processes, such as opening them using compatible software, importing data for analysis, and exporting data to different formats. These actions are crucial for accessing and using the information contained within STD files effectively.
Opening STD Files
Opening STD files requires specific software compatible with their format. For vector graphics templates, programs like Apache OpenOffice Draw are suitable as they are designed to work with this type of file. These programs use the STD file as a template for creating new drawings. In the context of semiconductor testing, specialized software that supports the Standard Test Data Format (STDF) is necessary to access the test information. One identified STD opener is compatible with this specific STDF type of file, enabling users to retrieve analysis results and other output data. It is important to note that different software may use files with the same .STD extension for different purposes, so selecting the correct program is crucial. Some programs may offer an import function, while others offer specific functions for opening this type of file. Furthermore, some STD files are plain text, and can be opened with editors such as Notepad.
Importing and Exporting STD Data
Importing and exporting STD data depends heavily on the specific STD file format being used. For STD files that function as vector graphic templates, the importing process typically involves loading the STD file into a compatible drawing program like Apache OpenOffice Draw. Once loaded, elements from the template can be used as a basis for creating new drawings. Exporting, conversely, could mean saving a newly generated drawing as an SXD file. When dealing with Standard Test Data Format (STDF) files, the process often involves using specialized software designed to handle semiconductor test information. These tools typically have specific options to import data from an STDF file and export data in various formats, including standard text files with comma-separated values. This way, data can be examined using a text editor or loaded into other software for further analysis. The format of the imported and exported data depends on the software.
STD and Programming
In programming, the ‘std’ namespace provides crucial tools for file operations, formatting, and result handling. Libraries like C++’s std⁚⁚format
and Rust’s std⁚⁚fs
and std⁚⁚io
facilitate these processes, allowing developers to interact with data effectively.
STD in C++ Formatting
The C++ standard library offers robust formatting capabilities through the std⁚⁚format
function, which allows developers to format data according to specified format strings. This functionality is crucial for generating human-readable output and for preparing data for storage or transmission. The std⁚⁚format
function takes format arguments and a format string, producing a formatted string as the result. Overloads are available for both character types, char
and wchar_t
, ensuring broad applicability across different character encodings. The formatting process may also incorporate locale-specific settings, allowing for proper handling of language and region-specific conventions. std⁚⁚format
is designed to be efficient and type-safe, preventing common errors associated with traditional formatting methods. When formatting, the result is usually copied to a newly constructed std⁚⁚string
. However, there are also overloads where the result is directed to an output iterator. Moreover, std⁚⁚vformat
is another function that serves a similar purpose but with slightly different requirements, specifically, its parameters do not require the constexpr
specifier. The std⁚⁚format
provides a significant improvement over older formatting techniques, making it easier and safer to handle string formatting in C++ applications.
STD in Rust File Operations
Rust’s standard library, often accessed through the `std` crate, provides comprehensive support for file operations using modules like `std⁚⁚fs` and `std⁚⁚io`. The `std⁚⁚fs⁚⁚File` structure is central to these operations, enabling the creation, reading, and writing of files. Writing to a file in Rust typically involves obtaining a file handle and using methods from the `std⁚⁚io⁚⁚Write` trait. Notably, the write operations in Rust can potentially fail, hence they return an `io⁚⁚Result`. This type represents either a successful write operation or an error, mandating that developers handle potential failure cases. This mechanism encourages writing robust code that does not ignore errors. The `std⁚⁚io` module also includes buffered writing through structures like `BufWriter`, which can enhance performance by reducing the frequency of system calls. Reading from files similarly uses `std⁚⁚io⁚⁚Read` trait and often involves `BufReader` for efficiency. Rust’s approach to file operations promotes both safety and performance, integrating error handling directly into the type system. The focus on explicit error handling and resource management makes file operations in Rust reliable and secure.
STD and Result Handling
In the context of programming, particularly with languages like Rust, `std` often relates to the standard library’s way of managing operations that might produce an outcome or an error. The `Result` type, prominently featured in the `std` crate, plays a crucial role in this. It is a generic enumeration representing the outcome of an operation; it is either `Ok(T)` with a successful value of type `T`, or `Err(E)` with an error value of type `E`. This explicit handling of success and failure is central to robust programming. In Rust, many functions that could fail, such as file I/O or network operations, return a `Result`, forcing the programmer to address both cases. This prevents silent errors and encourages a more resilient application design. The `std⁚⁚io⁚⁚Result` is a specific instance, often used for file operations, inheriting the base `Result` structure. The `Result` is a core concept for error handling in Rust, and promotes explicit error management. This approach contributes to writing safer, more reliable software. Result handling is fundamental when using the Rust standard library.